How to Optimize a Website by Eliminating Render-Blocking Resources
I recently embarked on a quest to optimise my website’s performance. Let me walk you through the techniques I employed and what was learned along the way.
What are Render-Blocking Resources?
Render-blocking resources are files that prevent a web page from loading quickly. These are typically CSS and JavaScript files that the browser must download, parse, and execute before it can render the page content.
Types of Render-Blocking Resources:
- CSS Files: Stylesheets that define the visual appearance of your page.
- JavaScript Files: Scripts that add functionality to your website.
- Web Fonts: Custom fonts loaded from external sources.
When a browser encounters these resources in the HTML, it pauses the rendering process until these files are downloaded and processed. Here’s a simplified example of how render-blocking resources appear in HTML:
html<head>
<link rel="stylesheet" href="styles.css">
<script src="script.js"></script>
</head>
<body>
<!-- Page content -->
</body>
In this example, both styles.css
and script.js
are render-blocking. The browser will wait to render the page content until these files are processed.
Why Eliminate Render-Blocking Resources?
Eliminating or optimizing render-blocking resources is crucial for several reasons:
- ๐Improved Page Load Speed: By reducing the time spent on these resources, your page loads faster.
- ๐Better User Experience: Faster-loading pages lead to higher user satisfaction and engagement.
- ๐SEO Benefits: Search engines, particularly Google, factor page speed into their ranking algorithms.
- ๐Lower Bounce Rates: Users are less likely to leave your site if pages load quickly.
- ๐ฑMobile Performance: On mobile devices with potentially slower connections, reducing render-blocking resources is even more critical.
To illustrate the impact, consider this scenario:
Without optimization:
Time to First Paint: 3.2s
Time to Interactive: 5.7s
After eliminating render-blocking resources:
Time to First Paint: 1.8s
Time to Interactive: 3.5s
These improvements can significantly enhance user experience and SEO performance.

Numerous studies support these findings, showing that even minor improvements in loading speed can greatly influence your website’s performance metrics.
Google and other search engines recognize that users favour fast-loading websites and aim to deliver the best search results. As a result, website performance has become a key ranking factor.
Google, in particular, has placed greater importance on user experience with the introduction of Core Web Vitals. Render-blocking resources can affect several of these metrics, including:
- Largest Contentful Paint (LCP)
- Total Blocking Time (TBT)
- First Contentful Paint (FCP)
- Interaction to Next Paint (INP)
The first three metrics alone account for 65% of the Lighthouse speed scoring system.

How to Find Problematic Files
Identifying render-blocking resources in WordPress involves a few key steps:
- Use Browser Developer Tools: Most modern browsers offer developer tools that can help identify render-blocking resources. In Chrome, you can:
- Right-click and select ‘Inspect‘
- Go to the ‘Network‘ tab
- Reload the page
- Look for files that are loaded early and block rendering
- Leverage Online Tools: These tools provide detailed reports on your site’s performance, including lists of render-blocking resources.
- WordPress-Specific Analysis: In WordPress, common culprits for render-blocking are:
- Theme CSS files
- Plugin CSS and JavaScript files
- Core WordPress files like
wp-includes/css/dist/block-library/style.min.css
- Use Query Monitor Plugin: This WordPress plugin provides in-depth analysis of page load, including:
- Enqueued scripts and styles
- Database queries
- Hooks and actions
Using PageSpeed Insights
The simplest method is to analyse your site using PageSpeed Insights. Just enter your website URL and initiate the analysis.
After completion, in addition to a wide range of data about your site’s performance, you’ll find the recommendations to eliminate render-blocking resources under the Diagnostics section.
PageSpeed Insights will also list the files responsible for slowing down your page loading time. That way, you know exactly where to start.
Here’s an example of what you might find in a PageSpeed Insights report:

Understanding these reports is crucial. They not only identify the problematic files but often suggest ways to optimize them.
Practical Steps to Analyse:
- Run your website through PageSpeed Insights.
- Note down all CSS and JavaScript files listed as render-blocking.
- Cross-reference these with your website theme and active plugins.
- Prioritize optimizing the largest files first, as they typically have the most significant impact.
By thoroughly understanding render-blocking resources and how to identify them, you’re well on your way to optimizing your website’s performance. Remember, the goal is not just to eliminate these resources blindly, but to optimize them in a way that balances performance with functionality and visual appeal.
In the next sections, we will explore strategies to optimize these resources, such as inlining critical CSS, deferring non-critical JavaScript, and optimizing web font loading. I’ve included links to further reading as each of these topics deserves its own in-depth exploration to truly master website performance optimization.
Optimized CSS Delivery
Removing Unused CSS
One of the first things I tackled was the bloat in my CSS files. I discovered that a significant portion of my stylesheets wasn’t being used at all. Here’s what I did:
- Used browser developer tools to identify unused CSS rules
- Manually removed unnecessary styles
- Employed tools like PurgeCSS to automatically strip unused CSS
Many web pages contain a large amount of unused code, particularly in the CSS. You can easily spot this by opening the Coverage tab in your browser’s developer tools.

It reveals the code that was loaded versus what was actually used to render the page, and the difference can be surprising.
This isnโt due to incompetence; it’s because stylesheets often include elements that donโt appear on every page, like forms or widgets, leading to unused markup. However, this unnecessary markup still consumes loading time and bandwidth, slowing down your site.
The first step is to remove code that isnโt needed. If itโs not being loaded, it canโt cause delays. Makes sense, right? You have two main ways to do this.
You can either inline critical CSS directly into the document head for faster loading or split your CSS files by screen size using the media attribute to load only what’s needed.
Tools like Sitelocity, PurgeCSS, or PostCSS can help automate this process.
The result is dramatically smaller CSS files, which means faster load times for visitors.
JavaScript Optimization
Deferred Loading and Delayed Execution for Render-Blocking JavaScript
JavaScript can be a major culprit in slowing down page loads. I implemented two key techniques:
- Deferred Loading: I added the
defer
attribute to my<script>
tags. This tells the browser to download the script while parsing HTML, but wait to execute it until the HTML is fully parsed. - Delayed Execution: For non-critical scripts, I used
setTimeout()
to delay their execution, ensuring that important content loads first.
async and defer
The two main tools you have for preventing scripts from delaying your pageโs from rendering are the async and defer attributes. You can add them to your calls in the code like so:
<script async src="important.js"></script>
<script defer src="also-important.js"></script>
Both attributes serve similar functions by preventing the browser from halting the page loading process when encountering JavaScript files, but they work in slightly different ways:
- async: The browser downloads JavaScript in the background and executes it as soon as it’s fully downloaded, without following the order in the document.
- defer: The browser also downloads the files in the background but waits to execute them until the entire page has loaded, while maintaining the original script order.
Here’s an illustration to clarify the difference.

These changes resulted in a noticeable improvement in my page’s initial load time.
Improving Font Loading
Fonts can significantly impact a site’s performance. I optimized my font loading strategy by:
- Using
font-display: swap
in my CSS to show a system font while custom fonts load - Preloading critical fonts with
<link rel="preload">
- Utilizing system fonts where possible to reduce the number of font files loaded
These tweaks ensured that my content was readable almost immediately, even on slower connections.
Caching and Compression
Implementing Browser Caching
Caching is like giving the browser a good memory. I set up browser caching by:
- Configuring my server to send appropriate caching headers
- Setting long expiry times for static assets like images and CSS files
This means returning visitors load my pages much faster, as their browsers remember parts of my site.
Compression Techniques
Compression is crucial for reducing the amount of data transferred. I implemented:
- Gzip compression: Enabled on my server to compress text-based files before sending them
- Image optimization: Used tools to compress images without significant quality loss
These methods substantially reduced my page size, leading to faster load times, especially on mobile networks.
Minification
Minification is about making file sizes smaller by removing unnecessary characters. I applied this to:
- HTML files
- CSS stylesheets
- JavaScript files
I used tools like UglifyJS for JavaScript and cssnano for CSS. The process removed all comments, whitespace, and formatted the code to be as compact as possible.
Additional Optimization Techniques
In addition to removing render-blocking resources, you can enhance your websiteโs performance through other methods:
- Caching: Saves static HTML copies of your pages on the server, reducing the need to dynamically generate them from PHP and the database for every visit.
- Image optimization: While not render-blocking, images significantly affect loading speed. Compressing images, using appropriate sizes, and adopting modern formats like WebP or Avif can improve load times, especially for mobile.
- Lazy loading: Loads images only when they appear on the screen, conserving bandwidth by delaying others until the user scrolls to them.
- Database optimization: Regular cleanup of redundant data in your database improves overall site performance.
- Content delivery network (CDN): A CDN distributes your static files across global servers, reducing load times by delivering them from locations closer to the user.
Results and Learnings
Here is the image of my website before any optimisations:

The performance score was low due to Render-Blocking Resources.
After removing unused CSS and JS, applying CSS, JS and HTML Minification, Font Display Optimisation, JS Defer for both external and inline JS, DNS Prefetch for static files and Gravatar Cache:
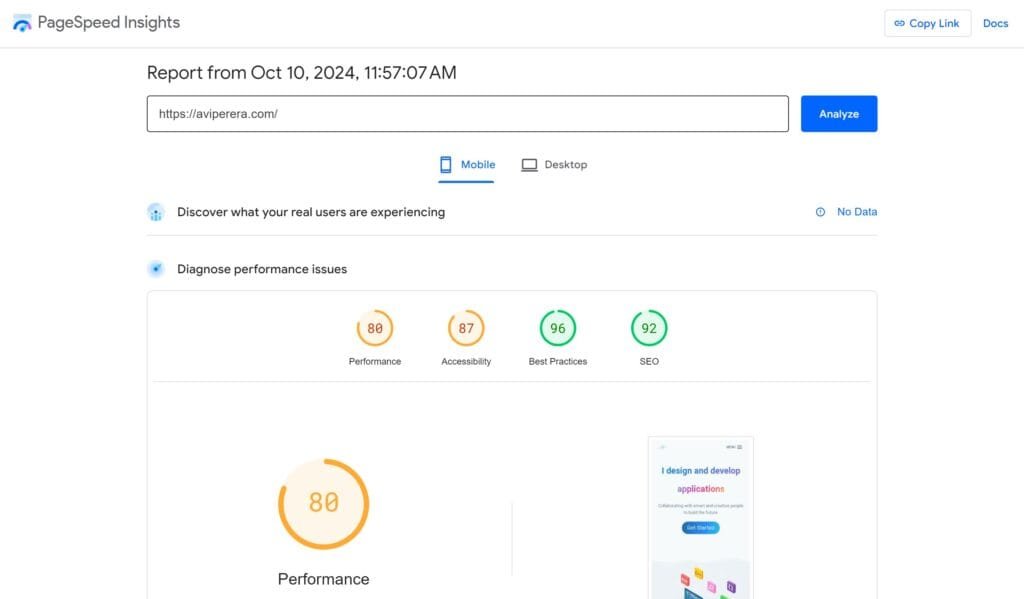
A significant improvement already!
I then went on to implement Lazy Load for Images, Viewport Image Generation, JS Delayed, Inline JS added to Combine, Inline CSS added to Combine, and a few other page optimizations:

After implementing these optimizations, I saw significant improvements:
- Page load times decreased by over 50%
- Mobile performance scores in Google PageSpeed Insights jumped from 69 to 94
- Bounce rates dropped, indicating visitors were more likely to stay and engage with my content
The process taught me the importance of continuous optimization and testing. It’s not just about implementing these techniques once, but regularly auditing and improving your site’s performance.
Remember, while these optimizations worked well for my website, every website is unique. It’s essential to test each change and measure its impact on your specific site.
By focusing on these key areas of web performance, you too can significantly improve your website’s speed and user experience. Happy optimizing!
If youโd like more tips on optimizing your website or are interested in booking a meeting to have me handle the process for you, feel free to get in touch through the contact page. Iโm always happy to help you improve your siteโs performance!

Avi is an International Relations scholar with expertise in science, technology and global policy. Member of the University of Cambridge, Avi’s knowledge spans key areas such as AI policy, international law, and the intersection of technology with global Affairs. He has contributed to several conferences and research projects, including collaborating with the United Nations Institute for Disarmament Research inaugural conference on AI, Security and Ethics.
Avi is passionate about exploring new cultures and technological advancements, sharing his insights through detailed articles, reviews, and research. His content helps readers stay informed, make smarter decisions, and find inspiration for their own journeys.
This was exactly what I needed. Thanks for the detailed post!
I’m glad that you found it useful! Please feel free to contact me if you have any questions. ๐